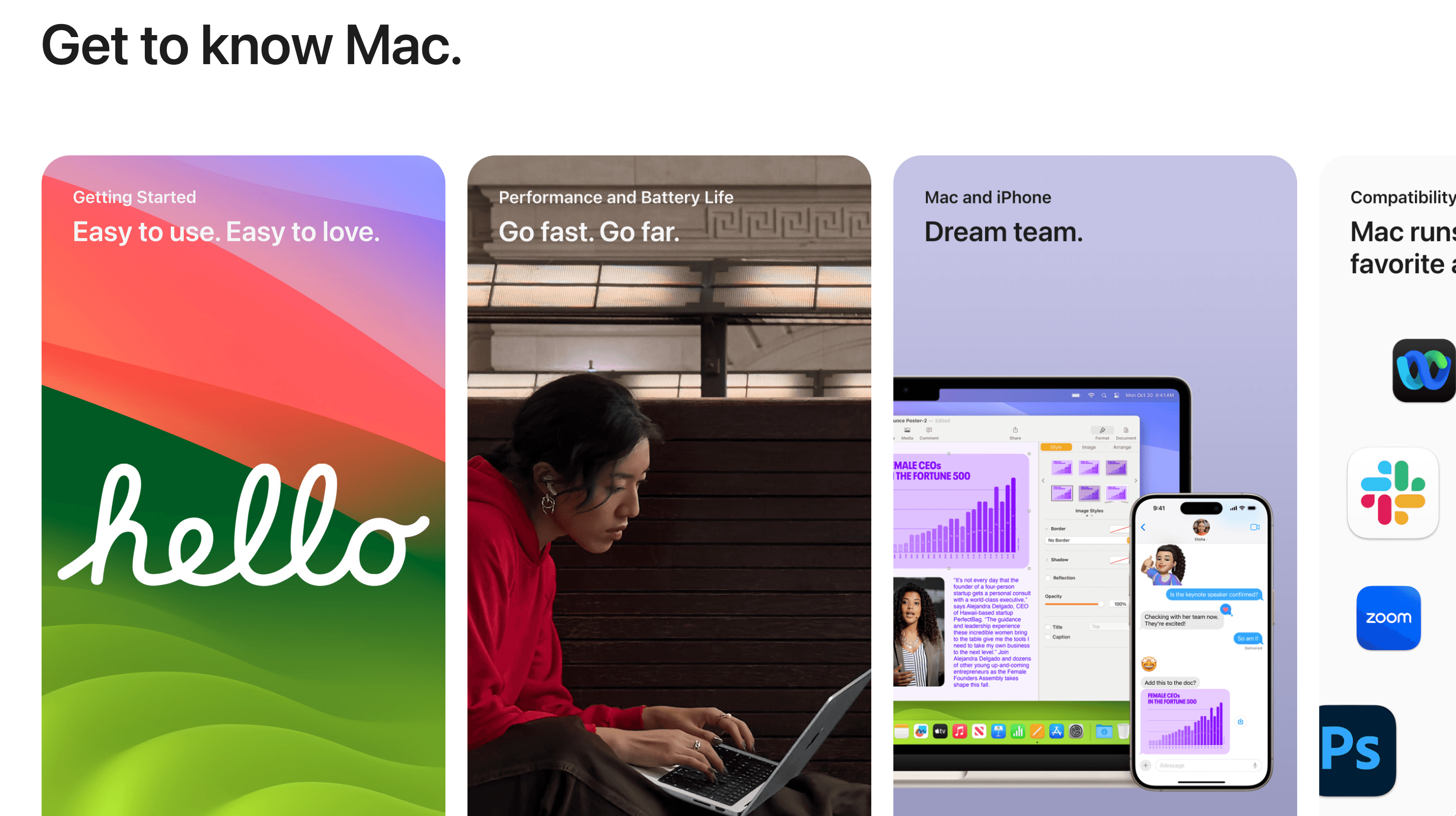
Animating content as it enters the viewport is a great way to showcase your site's content in an eye-catching way.
Popular brands like Apple have been doing it for years. The good news is it's never been easier to add this effect to your own site.
Follow these simple steps and you'll be animating in no time!
Step 1: Define CSS Animations
Start by creating our first animation, a "fade up" effect.
If you're using Next.js, you'll add these to your global.css
file.
@keyframes fadeUp {
from {
transform: translateY(100px);
opacity: 0;
}
to {
transform: translateY(0);
opacity: 1;
}
}
.animated {
opacity: 0;
animation-duration: 1s;
animation-fill-mode: both;
}
.fadeUp {
animation-name: fadeUp;
}
Step 2: Install React Intersection Observer
This is a helpful React hook that provides a ref
(i.e. "reference") to the element you want to observe and a boolean, InView
, that let's you know the whether or not the element is currently visible to the viewer.
npm i react-intersection-observer
Step 3: Create an Animate In View Component
"use client" // Include this if you're site is built with Next.js
import { useInView } from "react-intersection-observer"
export default function AnimateInView({ children, transition }) {
const { ref, inView } = useInView({
triggerOnce: true, // Set to false if you want animation to reoccur with visibility changes
});
return (
<div ref={ref} className={animated ${inView ? transition : ""}
}>
{children}
</div>
)
}
Step 4: Use the AnimateInView Component
The AnimateInView
component will apply the animation to all child components.
import AnimateInView from "@/app/components/AnimateInView"
export default function Page() {
return (
<AnimateInView transition="fadeUp">
<h1>Heading that I want to animate</h1>
<p>paragraph that I want to animate</p>
</AnimateInView>
)
}
The output for this code should render a page on your site that looks similar to what you see below. I created a page on my site for demo purposes.
That's it! You now know how to animate in view.
Step 5: Advanced Animations
To demonstrate, here is an example of how I'm currently using this very AnimateInView
component on my own site. I added some placeholder posts for demo purposes.
You can get creative with the animations. For instance, to animate in from the right, add the following to your CSS file.
@keyframes fadeInRight {
from {
transform: translateX(100%);
-webkit-transform: translateX(100%);
filter: blur(10px);
}
to {
transform: translateX(0);
-webkit-transform: translateX(0);
filter: blur(0);
opacity: 1;
}
}
.fadeInRight {
animation-name: fadeInRight;
}
Then, just pass the appropriate animation class to your AnimateInView
component.
export default function Page() {
return (
<AnimateInView transition="fadeInRight">
<h1>Heading that I want to animate from right</h1>
<p>paragraph that I want to animate from right</p>
</AnimateInView>
)
}
Conclusion
If you liked this post, be sure to subscribe to my newsletter below to receive future posts.
Animate In View Library
If you're looking for a dead simple drop-in solution to immediately add these animations and more to your site, check out my latest product, Animate In View!